Syntax Error #3: Who let the ducks out?
This time, the elephant in the room is actually a duck! Thankfully, this is not a zoology newsletter so dive in to see how the ducks can help you out.
Do you sometimes wish your bugs would just be April's Fool jokes and you didn't have to fix them? I sometimes do. But until that happens, here's the third issue of Syntax Error newsletter to help you debug the bugs you encounter.
The Rubber Duck
We made it to issue 3 of a debugging newsletter before actually talking about the ducks.
If you've been on the industry for a while, you may have heard discussion about rubber ducks, seen some on your colleagues desks or have stickers on your laptop with them. They are a lovely part of the developer culture but they are more than just an inside joke.
A quick primer on rubber ducks for those unfamiliar with the concept:
The idea with rubber duck debugging is that when you face a problem that you can't solve, you pick up a duck and ask help from it. To get help from a duck, you need to explain the problem, the steps you've taken, what you think might be the issue – just like you would with a colleague. And often, before the duck has time to answer, you've realized what the issue is and how to solve it.
The science behind it is, that when you need to explain your problems, you think about them in more structured and detailed way and you start to notice missing links, misconceptions and mistakes.
A duck is also a great sparring partner to help you become better at asking questions. Asking better questions makes you also better at writing bug tickets and helping others with their problems. If you want to maximize the quality of an answer from anyone, I recommend reading this blog post about how to ask help for technical problems.
Sometimes, you are so deep into your assumptions and blurred knowledge of the situation that it's hard to mentally remove yourself from the situation. When that happens, I recommend doing a "brain dump" - writing down everything you know or assume about the situation - and going for a short walk or taking a nap to give your mind other things to think about.
A few ducks in my life
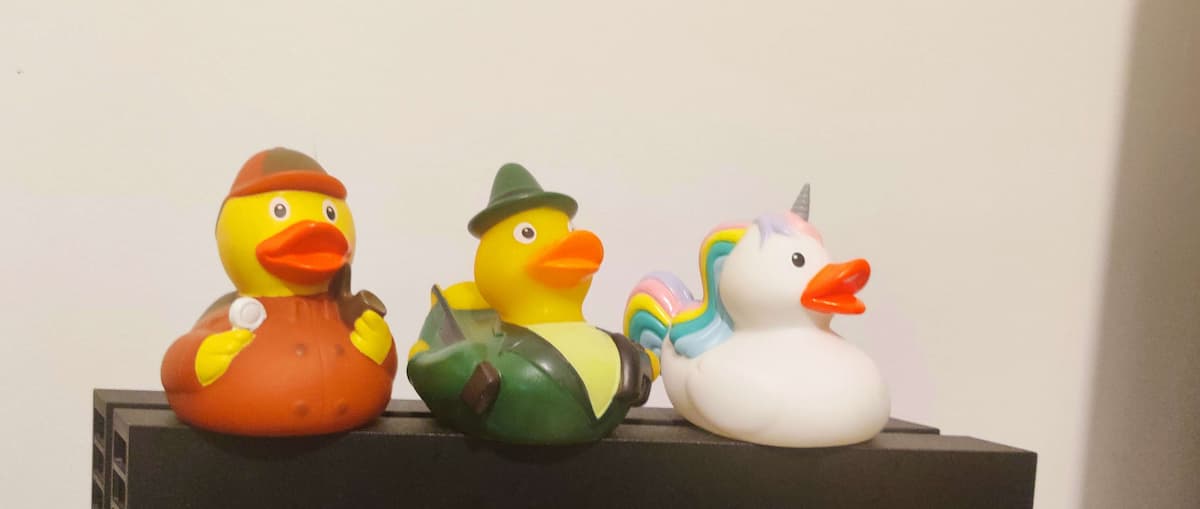
The most common duck is a yellow bath duck but there are hundreds of more, specialized in different skills. My current crew consists of Sherlock Rub-A-Duck, a mastermind detective; Frank, who can spot bugs from far away with their binoculars and Uni, who's got magical powers to heal bug wounds.
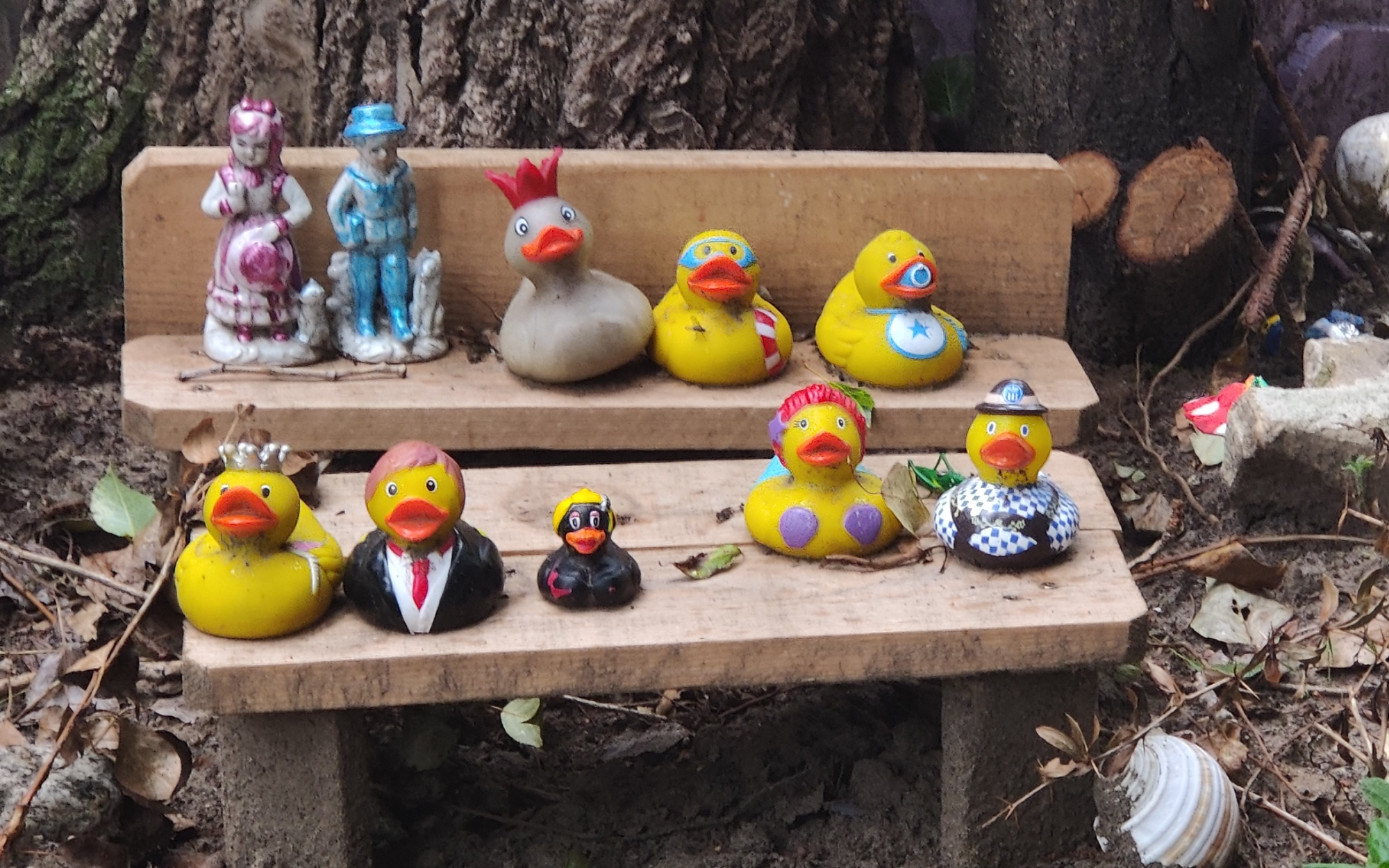
When I lived in Berlin, Germany, I also had access to the Council of Ducks in my neighborhood. It was a very diverse group of ducks who had seen a lot so when my own crew wasn't enough, I trekked to the Tree of Wisdom and asked for a session with the Council.
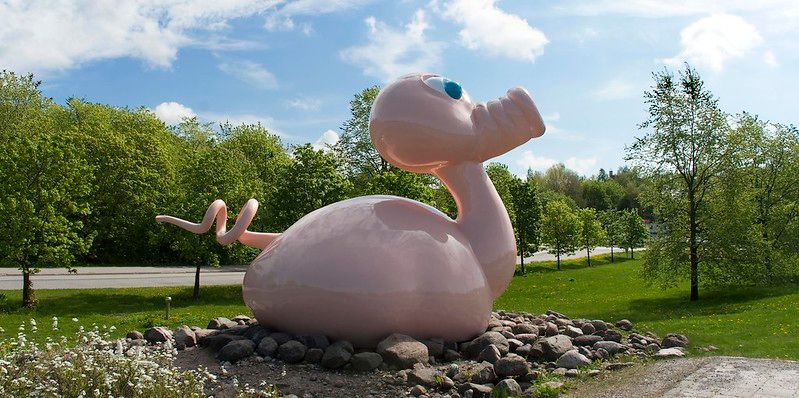
In my current hometown of Turku, Finland, there's another option too. You can ask help from Posankka, an art piece by Alvar Gullichsen that is 5 meters high and combines a pig and duck and is told to have great debugging powers.
Sometimes, you are on the road away from your usual duck crew and might not have access to ducks. In that case, you can ask remote help from Cyberduck or Duckie who are always willing to lend a foot. A wing? What's the equivalent of hand with ducks?
Send me pictures of your ducks (hello@syntaxerror.tech or toot them at me @hamatti@hamatti.org) and I'll make a collage for a future issue of Syntax Error!
Stack trace of the Month
This month's error section focuses on Rust and two of the features/tools specifically: borrow checker and rustlings.
Rust's borrow checker
Rust's ownership system is one of the most defining and unique features of the language. It enables Rust to operate in a memory-safe way without a garbace collector. However, you don't need to fully understand it to see the beauty in the borrow checker from a developer's perspective.
Let's start with a code snippet from Rust book:
fn main() {
let s = String::from("hello");
change(&s);
}
fn change(some_string: &String) {
some_string.push_str(", world");
}
When we attempt to compile it, we'll get a very nice error output:
$ cargo run
Compiling ownership v0.1.0 (file:///projects/ownership)
error[E0596]: cannot borrow `*some_string` as mutable, as it is behind a `&` reference
--> src/main.rs:8:5
|
7 | fn change(some_string: &String) {
| ------- help: consider changing this to be a mutable reference: `&mut String`
8 | some_string.push_str(", world");
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ `some_string` is a `&` reference, so the data it refers to cannot be borrowed as mutable
For more information about this error, try `rustc --explain E0596`.
error: could not compile `ownership` due to previous error
The borrow checker notices that you are trying to do something that is not allowed and lets you know. But even better than just letting you know, it also gives you tips for how to fix it ("help: consider changing this to be a mutable reference &mut String") and it gives a command (rustc --explain E0596
) that you can run to get more information about the issue. So let's run it!
This error occurs because you tried to mutably borrow a non-mutable variable.
Erroneous code example:
```
let x = 1;
let y = &mut x; // error: cannot borrow mutably
```
In here, `x` isn't mutable, so when we try to mutably borrow it in `y`, it
fails. To fix this error, you need to make `x` mutable:
```
let mut x = 1;
let y = &mut x; // ok!
```
rustc --explain E0596
This is simply fantastic. You get so much information directly in your terminal: what is the problem, where to learn more and even suggestions for how to fix it. Often that's enough to give you pointers to fix it without having to open a search engine or sending a message to a colleague or discussion forum.
rustlings
Rustlings is a project that helps new developers learn Rust through reading compiler errors and fixing them through exercises. I love that project and have written a blog post and a talk about it for Rust Finland meetup in May, 2022.
After you install rustlings and run it with rustlings watch
, you'll get a compiler error:
⚠️ Compiling of exercises/variables/variables1.rs failed! Please try again. Here's the output:
error[E0425]: cannot find value `x` in this scope
--> exercises/variables/variables1.rs:12:5
|
12 | x = 5;
| ^ not found in this scope
error[E0425]: cannot find value `x` in this scope
--> exercises/variables/variables1.rs:13:36
|
13 | println!("x has the value {}", x);
| ^ not found in this scope
error: aborting due to 2 previous errors
For more information about this error, try `rustc --explain E0425`.
Type 'hint' or open the corresponding README.md file to get help or type 'clear' to clear the screen.
rustlings watch
on a brand new rustlings installationIt will show you the actual error and it's your job to learn how to read it, find the right file, change the code and see if the compiler becomes happier.
I've seen other projects in other languages that provide failing test cases where you need to fix the code but this was the first one for me that uses compiler errors in such a beautiful way. It's not perfect and you can cheat by just changing the code to anything that compiles but if you're out there learning a new language, why would you cheat yourself?
Story of the Month
If you have debugging stories or examples you want to share to the Syntax Error community, please reach out via hello@syntaxerror.tech and we might share your story!
This month's story is not directly from a reader but it comes from djinn and juice blog, from a post titled "The Best Debugging Story I’ve Ever Heard". How could we skip a story with such a juicy title?
This story takes us back in the time, to a different age into the 80s when there weren't SSDs or USB drives and all that jazz but data was being stored to tape.
One customer had a problem. In the middle of a print run, one particular A drive would stop working, causing the entire print run to stop. To restore the drive the attendants had to reboot the entire drive - and if this happened in the middle of a six-hour print job, there’d be a ton of expensive computer time lost and the whole operation would fall behind schedule.
Debugging software issues can be challenging but can you imagine facing an issue like this? Every time you want to run it, you'd need to wait for (and waste) hours of time and expensive equipment just to see it crash over and over again. I'm starting to like the printing technique from last month's issue more and more.
The Expert sat down in his chair again, waiting for it to crash. It took something like six hours of waiting, but it crashed again. He still had no idea what was causing it, other than the fact that it happened when the room was crowded. He ordered that the job be restarted, and he sat down again and waited.
Imagine the self-confidence of this expert. Asking over and over again for the client to run the long and expensive operation without even making any changes mid-way. I admit that I might have had a bit of sweat on my forehead at that point.
But the story gets even better with the solution.
The crash occurred when the attendants were changing the tapes on an unrelated drive. And furthermore, he realized that the crash occurred as soon as one of the attendants walked across a certain tile on the floor.
[ – ]
The Expert figured out that one of the aluminum tiles was warped. When an attendant stood on the corner of the warped tile, the edges of the tiles rubbed together. As the plastic connecting the tiles rubbed together, they produced microsparks, which in turn caused RF interference.
This sure makes my Python typos feel more feasible bugs to approach.
I recommend reading the entire story in the blog, it's a great piece of debugging history.
Syntax Error is created with love by Juhis. If you liked it, why not share it with a friend? Or if you have any feedback or just want to say hi, hit reply. I'm always happy to hear from my readers and learn about what you do and how you debug your issues.