Syntax Error #7
Hello!
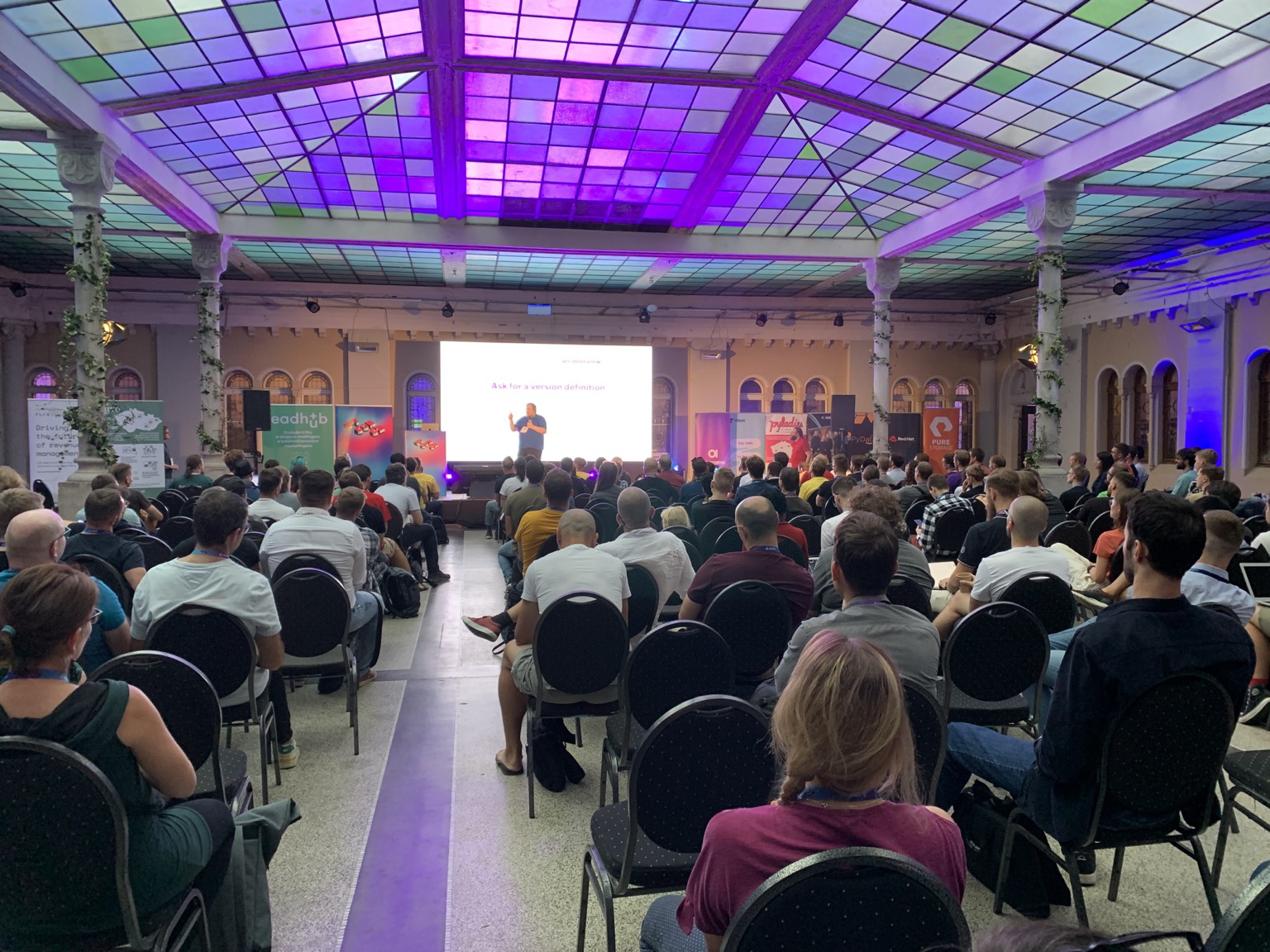
As I'm sending this newsletter, I'm enjoying the lovely Czech Python community at PyCon CZ. Prague has been beautiful as always and I got the pleasure to entertain the audience with my lightning talk about the wild west of version numbering schemes.
If you're working with Python and want to experience the best, most friendly and loveliest and warmest conference, I wanna recommend joining PyCon CZ next year. I've been here twice and both times have been fantastic.
Javascript bug and its debug
Let's start this month with a bit of role play: imagine that you are a web developer working in an educational institute and you are part of a team that's responsible for the part of code that grades the student's course work to be displayed in online protal and their certificates.
It's a bit of legacy system but things have been working okay. You get the scores for a student per course from an API developed by a third party vendor.
Then one day, all hell breaks lose. Students start to report through their teachers and administration that the grades are all wrong and some of them are worried it will cost them their future opportunities. The grades are still reported but they are bit inconsistently incorrect: sometimes they get some students right and sometimes they may fail a student who would have otherwise gotten a high grade.
That's a stressful situation to be.
Let's debug!
In a situation like this, my first approach is to start from the end user's perspective: in this case the web portal. If I'm not sure what part of the system is responsible for what calculations, I'd start by logging in and seeing what API calls are being made and what they return.
In Firefox, I'd do this by opening the Dev Tools and heading over to the Network tab. I find the right call from the list (I discover that frontend calls endpoint /grades
) and from the Response tab on the right pane, I can see that the backend is sending us the final grades.
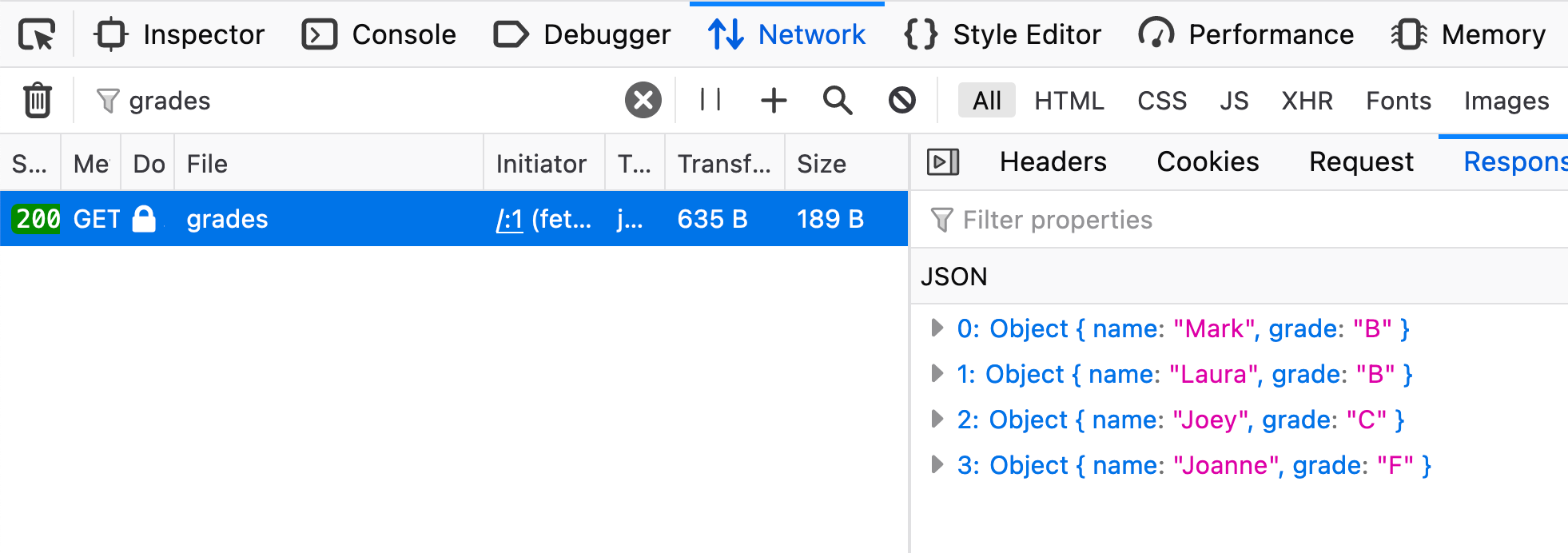
This helps me divide the problem space in half: I know the error is not in the frontend code. That's why I always start with this step as it saves a lot of time.
Moving to backend
As I learned through exploration in the last step that my code calls the endpoint /grades
, my next step is to open the routing files in the backend code to see what happens when /grades
gets a GET request.
Following through a few hoops, I find that we have a getGrades
function that is responsible for grading.
function grade(score) {
if (score > 70) return 'A';
if (score > 50) return 'B';
if (score > 30) return 'C';
if (score > 10) return 'D';
return 'F';
}
async function getGrades(student, course) {
const scoresFromAPI = await getScores(student, course);
const scores = scoresFromAPI.map(parseInt);
const total = calculateTotal(scores);
const grade = grade(total);
return grade;
}
On a quick glance, everything seems normal: we get the scores as strings (remember how I said we use 3rd party APIs, you sometimes find funky stuff like this in real life) and we convert them to integers with Javascript's parseInt function, then calculate the total score and finally get the grade and return that.
(If you're an experienced Javascript developer or have been burned by this recently, you might already know the issue. Play along, don't spoil it for the crowd.)
I'd start by adding a console.log
, my first tool in the toolbox for every debugging into the function. First, to make sure it's being run through here and second, to see what we get.
async function getGrades(student, course) {
const scoresFromAPI = await getScores(student, course);
console.log({ scoresFromAPI })
const scores = scoresFromAPI.map(parseInt);
const total = calculateTotal(scores);
const grade = grade(total);
return grade;
}
When I now refresh the dev environment, I can see that the scores are indeed coming in:
{
scoresFromAPI: ["14", "10", "13", "10"]
}
Good, now we've confirmed that we are in the right place (and we're in the right environment).
Next, maybe I want to make sure they get parsed correctly:
async function getGrades(student, course) {
const scoresFromAPI = await getScores(student, course);
const scores = scoresFromAPI.map(score => {
let parsed = parseInt(score)
console.log(parsed)
return parsed
});
const total = calculateTotal(scores);
const grade = grade(total);
return grade;
}
14
10
13
10
Once again, everything looks to be in order, hmm. I then go refresh my page again and I notice now the grades have changed a bit, that's odd. All I did was add some logging statements with no side effects but the results are different.
You roll back that change and confirm that things are broken again. Sometimes one just gets stuck.
Becoming a code historian
History is a good teacher. I often use version control's history to see what recent changes may have happened here that could explain.
With the help of git blame
and following the trail of changes within this function, you find a commit where it was changed:
async function getGrades(student, course) {
- const scores = await getScores(student, course);
+ const scoresFromAPI = await getScores(student, course);
+ const scores = scoresFromAPI.map(parseInt);
const total = calculateTotal(scores);
const grade = grade(total);
return grade;
}
The explanation tells you that the API for some mysterious reason changed from returning a list of integers to returning a list of strings so the developer who made the change, added a map function that parses them to integers.
But we checked that and those scores were parsed correctly – at least when we printed them out.
Here's a short Javascript lesson: the functions that you pass as callbacks to other functions like map
and filter
need to have a specific signature or they can fail horribly. Jake Archibald has a great blog post going in depth with this.
In essence, the callback function provided to map
, is passed three arguments: the element, its index and the array it came from. On the other hand, parseInt takes in two arguments: the number and its base.
So what happens here is that we actually call parseInt
with values (14, 0), (10, 1), (13, 2) and (10, 3)
so instead of converting them into integers base 10 (decimal), we try to convert numbers into base 0, 1, 2 and 3 - causing them to fail in a way that still often returns a number.
And what you as the developer in this role play didn't either look into or consider anymore is that the calculateTotal
function converted any NaN
s to 0 because sometimes missing scores were inregularly marked in the source data.
Happy end
We started the debugging journey from the browser, found our way through network requests and routes to our function and finally after a while of bouncing around, found a helpful hint from the git history that allowed us to fix the code.
The fix? To change the map line into const scores = scoresFromAPI.map(score => parseInt(score))
which so easily looks to do the same thing when it actually doesn't.
Story Time
If you have debugging stories or examples you want to share to the Syntax Error community, please reach out via hello@syntaxerror.tech and we might share your story!
I ran into this interesting post A Taxonomy of Bugs the other day. Niklas Gray wrote an interesting categorization of different types of bugs. He categorized them as:
- The Logical Error
- The Unexpected Initial Condition
- The Memory Leak
- The Memory Overwrite
- The Design Flaw
- The Third-Party Bug
- The Failed Specification
- The Hard-To-Reproduce Bug
- The Compiler Bug
He talks about each of these cases and I really like that he included things that might not traditionally be considered bugs but definitely have similar outcomes like design flaws and failed specs.
Head over to https://ruby0x1.github.io/machinery_blog_archive/post/a-taxonomy-of-bugs/index.html and give it a read!
Syntax Error is created with love by Juhis. If you liked it, why not share it with a friend? Or if you have any feedback or just want to say hi, hit reply. I'm always happy to hear from my readers and learn about what you do and how you debug your issues.